在网上看到一个平滑算法,很好用,经过测试它的效率很高,Chaikin 函数调用2-3次会获得一个相对较好的曲线。算法链接
原理是不断的裁切三角形让其分裂成2个三角,2个三角变成4个,以此类推,最终会越来越平滑从而接近一个圆。
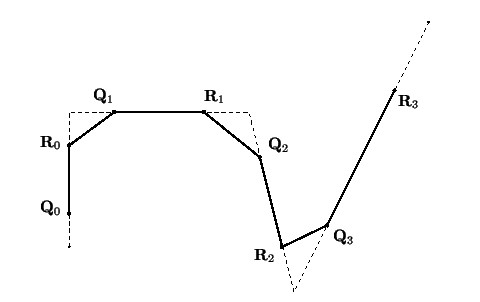
平滑算法如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
| using UnityEngine; using System.Collections;
[RequireComponent(typeof(LineRenderer))] public class SmoothAlgorithm : MonoBehaviour {
private float minRadius = 1.5f; private float maxRadius = 3.5f; private int num = 25; private LineRenderer lr; private Vector3[] points;
void Start() { lr = GetComponent<LineRenderer>(); GenerateRandomPath(); SetLR(points); }
void GenerateRandomPath() { points = new Vector3[num];
float angle = 360.0f / num;
for (int i = 0; i < num; i++) { points[i] = Quaternion.AngleAxis((angle * i), Vector3.forward) * Vector3.up * Random.Range(minRadius, maxRadius); } }
void SetLR(Vector3[] pts) { lr.SetVertexCount(pts.Length); for (int i = 0; i < pts.Length; i++) { lr.SetPosition(i, pts[i]); } }
void Update() { if (Input.GetKeyDown(KeyCode.Space)) { points = Chaikin(points); SetLR(points); }
if (Input.GetKeyDown(KeyCode.G)) { GenerateRandomPath(); SetLR(points); } }
Vector3[] Chaikin(Vector3[] pts) { Vector3[] newPts = new Vector3[(pts.Length - 2) * 2 + 2]; newPts[0] = pts[0]; newPts[newPts.Length - 1] = pts[pts.Length - 1];
int j = 1; for (int i = 0; i < pts.Length - 2; i++) { newPts[j] = pts[i] + (pts[i + 1] - pts[i]) * 0.75f; newPts[j + 1] = pts[i + 1] + (pts[i + 2] - pts[i + 1]) * 0.25f; j += 2; } return newPts; } }
|
本文标题:三维向量曲线平滑算法
文章作者:Keyle
发布时间:2017-12-22
最后更新:2024-08-20
原始链接:https://vrast.cn/posts/7a035f20/
版权声明:©Keyle's Blog. 本站采用署名-非商业性使用-相同方式共享 4.0 国际进行许可